Unreal Material Graph Connector Colors
Here is a quick post with a small code change to randomly color material graph connectors in Unreal.
Sometimes I can find it hard to navigate material node graphs in Unreal, particularly as the number of connections increases.
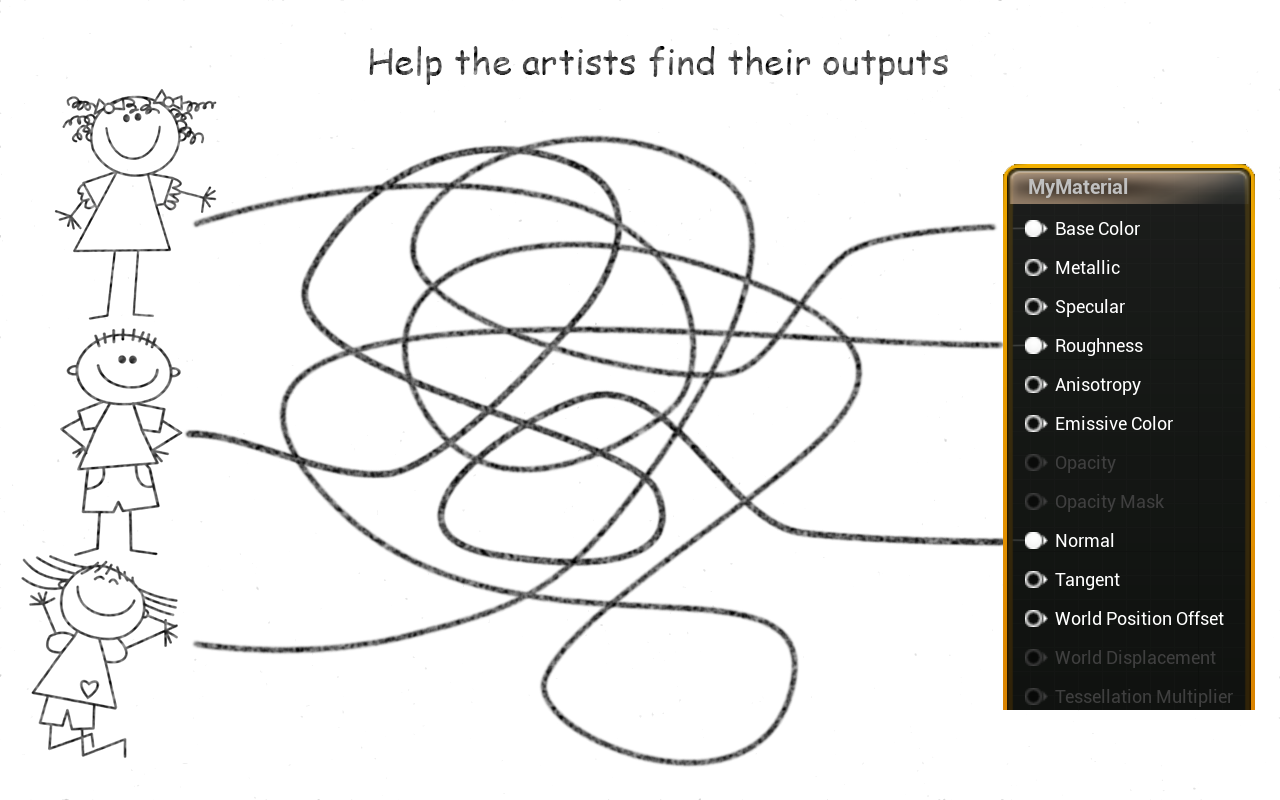
For other node graphs types in Unreal the connector colors can be based on the type of data but in a material they are all displayed as white.
Here is a little engine code hack I use locally that I find useful. It basically gives each connector in the material graph a random color based on the hash of the input and output pin.
Example Material Graph Without the Code Change
Example Material Graph With the Code Change
There is already an option “Hide Unrelated” that will draw unselected nodes and connectors transparent but I still find having the code change below enabled is generally useful.
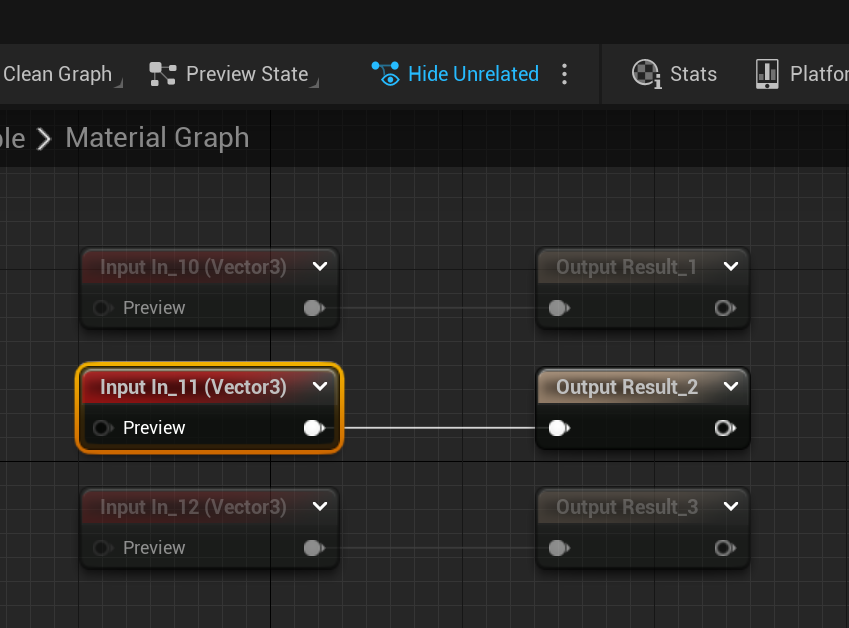
'Hide Unrelated' Option Enabled
There may well be alternative ways to achieve this. It wont be the first time I implement something in Unreal Engine only to find there is a CVar that already implements the feature.
The code isn’t particularly clever, the color you get is somewhat arbitrary but the size of the engine change is pretty small and I’ve found it useful (and added this for the whole team) on a number of projects.
Applying the change
You will want to search for the function: FMaterialGraphConnectionDrawingPolicy::DetermineWiringStyle()
and copy and paste the snippet of code below. Then recompile the editor.
void FMaterialGraphConnectionDrawingPolicy::DetermineWiringStyle(UEdGraphPin* OutputPin, UEdGraphPin* InputPin, /*inout*/ FConnectionParams& Params)
{
Params.AssociatedPin1 = OutputPin;
Params.AssociatedPin2 = InputPin;
Params.WireColor = MaterialGraphSchema->ActivePinColor;
// -------- 8< -------- 8< -------- 8< -------- 8< -------- 8< -------- 8< --------
// Begin Change to Assign Random Colour to Material Graph Connections
#if 1
// Color the connector based on the hash of the InputPin and OutputPin
if (OutputPin && InputPin)
{
const uint32 hash32Input = FCrc::MemCrc32( &InputPin->PinId, sizeof(FGuid) );
const uint32 hash32 = FCrc::MemCrc32( &OutputPin->PinId, sizeof(FGuid), hash32Input );
const uint8 hue = static_cast<uint8>( hash32 & 0xff );
const uint8 saturation = 192; // 0 = desaturated, 255 = fully saturated. We use < 255 as we want generally lighter colours
const uint8 value = 255;
Params.WireColor = FLinearColor::MakeFromHSV8( hue, saturation, value );
}
#endif
// End Change to Assign Random Colour to Material Graph Connections
// -------- 8< -------- 8< -------- 8< -------- 8< -------- 8< -------- 8< --------
And that’s all!
This change worked in UE4.26 and the early access UE5 as of June 2021. Of course there may be other issues so use at your own risk.
Hopefully this may help a little navigating around the more complex material graphs. This is not a substitute for planning and organising your materials, neatly layout out your graph nodes and sensible use of material functions, comments and reroute nodes!